Using pre-trained models
As an alternative to training your own models you can use one of the pre-trained models available. SentiSight.ai currently offers eight pre-trained models - General classification, Places classification, NSFW classification, Object detection, Instance segmentation, Text recognition, Pose estimation and Background removal. You can see the list of categories included in General classification model here, categories included in Places classification here categories included in Object detection model here and Instance segmentation model here. NSFW classification has only two categories "safe" and "unsafe" that simply tells you whether or not an image contains adult content. Text recognition model marks the location of the text in an image with bounding boxes similarly as in object detection and returns parsed text pieces. The Pose estimation model marks the location of detected people in an image together with keypoints, such as head, shoulder, elbow, wrist, hip, knee, ankle, for each detection. Lastly, the Background removal model detects the primary object and the background in an image and erases the background.
There are two main options to use a pre-trained model: via SentiSight.ai web platform or via REST API.
Using pre-trained models via SentiSight.ai web platform
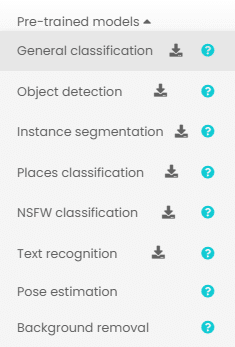
To use a pre-trained model click on "Pre-trained models" dropdown on the top panel of the main dashboard, then choose one of the models in the list. This will take you to the prediction screen and let you select images to upload from your computer.
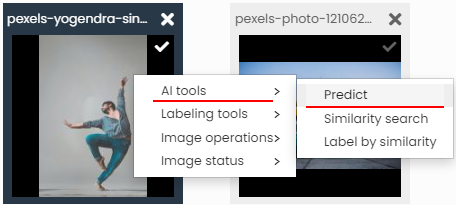
Alternatively, you can launch predictions on existing project images.
Right-click an image in the project, click Predict and then choose desired model.
This will open predictions window and immediately launch prediction with selected model.
General classification, NSFW and Places predictions are a form of classification predictions. Beside the image is a list of labels that the image may belong to, with percentage assigned to each label, showing the algorithm's confidence in that particular label being present in the image.
Places and General classification models usually suggest multiple labels, while NSFW only operates with two labels - safe and unsafe.
Prediction results can be downloaded either in CSV or JSON format, or as a ZIP with query images grouped into folders by predicted labels.
You can read more about image classification and classification predictions here.
General classification
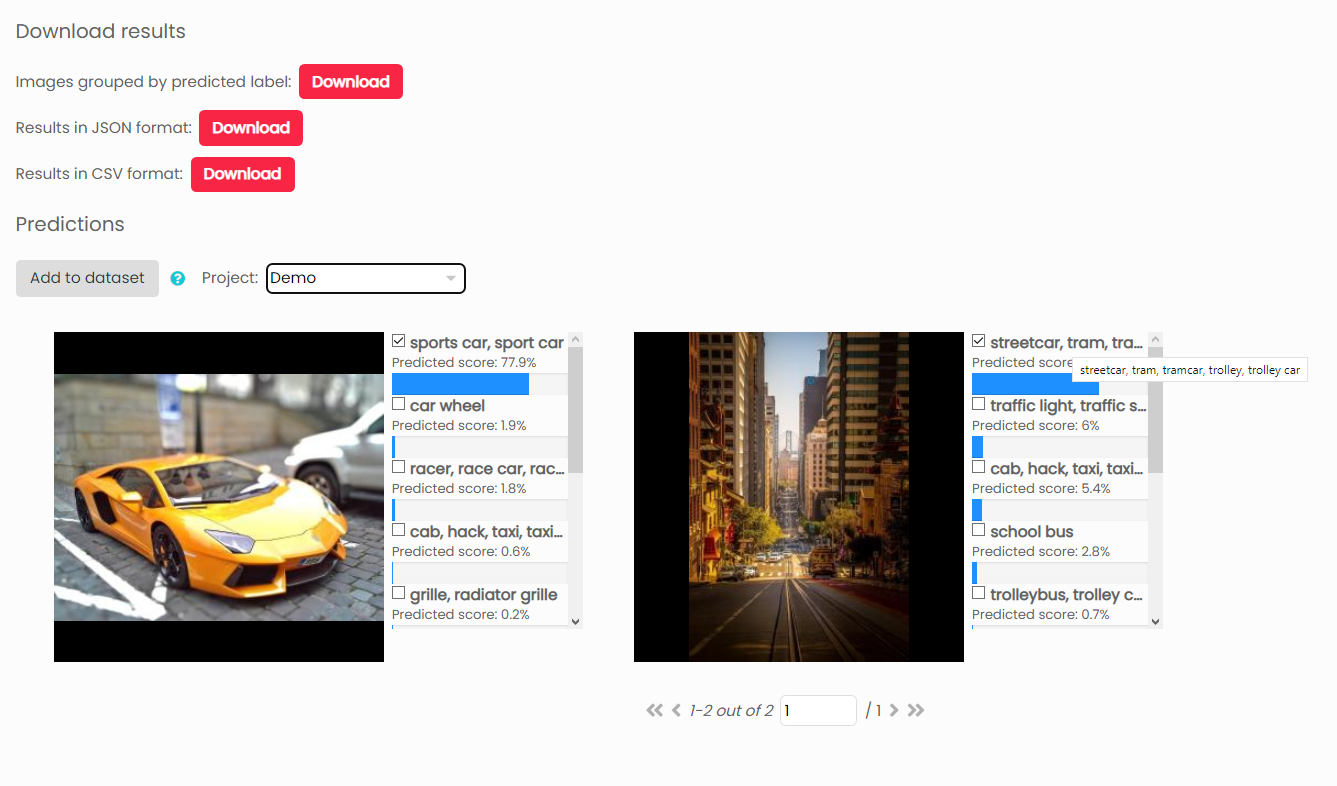
Places classification
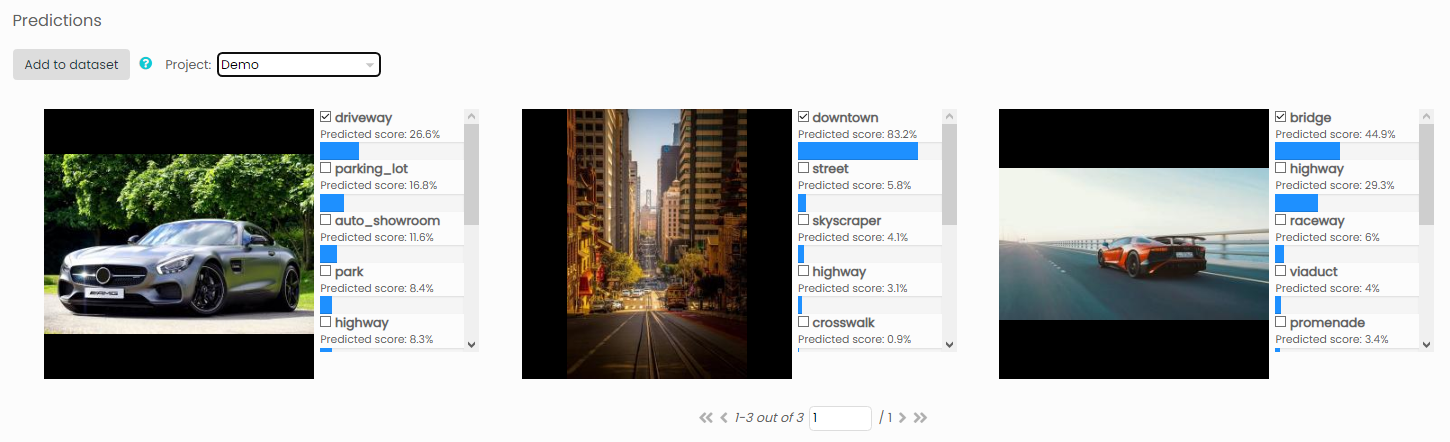
NSFW classification
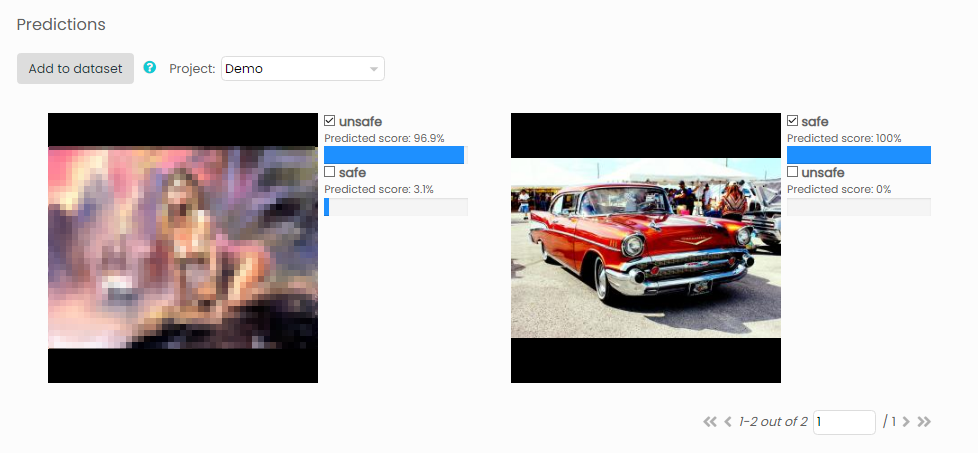
Object detection predictions draw a bounding box around the parts of the image that contain the predicted label. They can be downloaded either in JSON format or in a ZIP file containing query images with bounding boxes drawn on them.
You can read more about object detection predictions here.
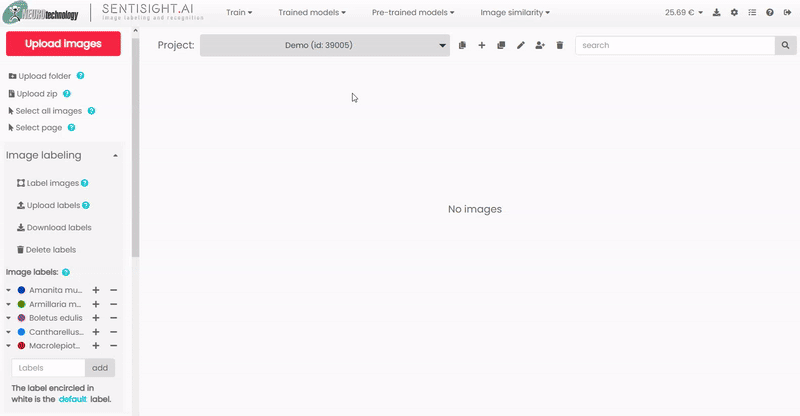
Instance segmentation predictions precisely cover the parts of the image that contain the predicted labels with a bitmap mask. They can be downloaded either in JSON format or in a ZIP file containing query images and matching bitmaps.
You can read more about Instance segmentation predicitons here.
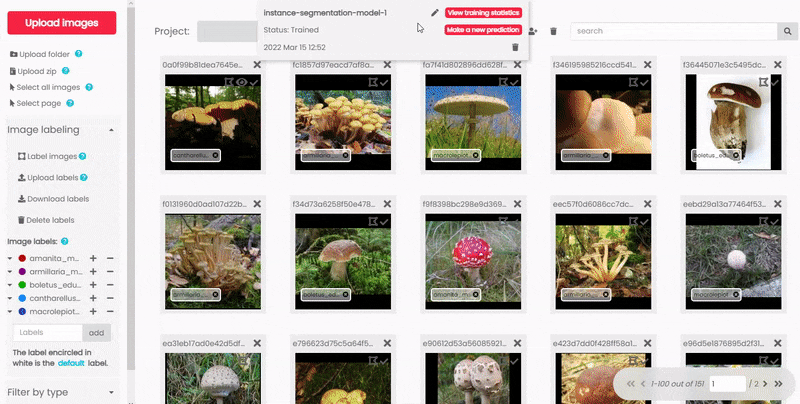
Pose estimation predictions take form of a set of connected keypoints showing main joins and shape of a human body.
They can be downloaded either in JSON format or a ZIP file containing query images with pose keypoints and lines drawn on them.
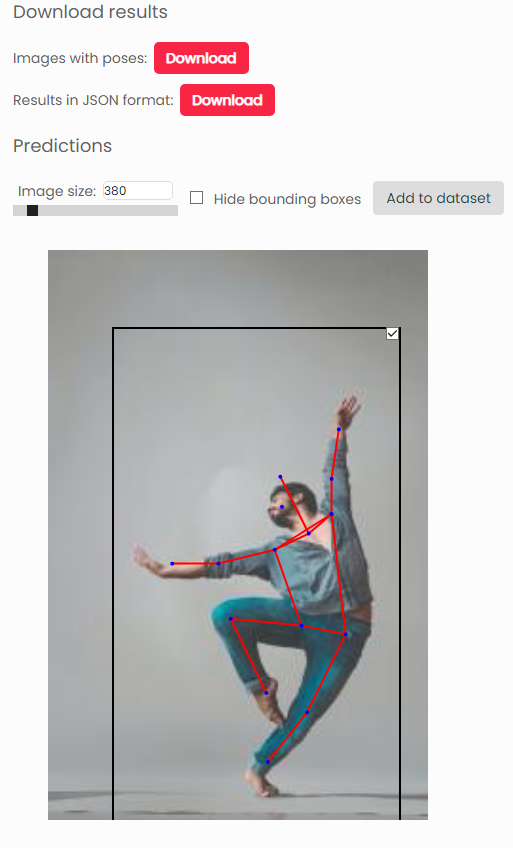
Text recognition predictions are two-fold. First, you have parts of the query image that the algorithm found text in highlighted with bounding boxes and prediction confidence percentage. Then, you have a transcript of all text detected in below the image, so it is easier to read.
You can download text recognition predictions in JSON format.
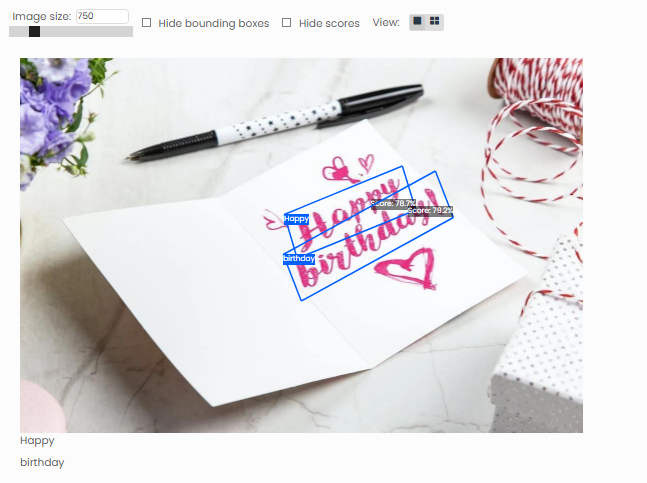
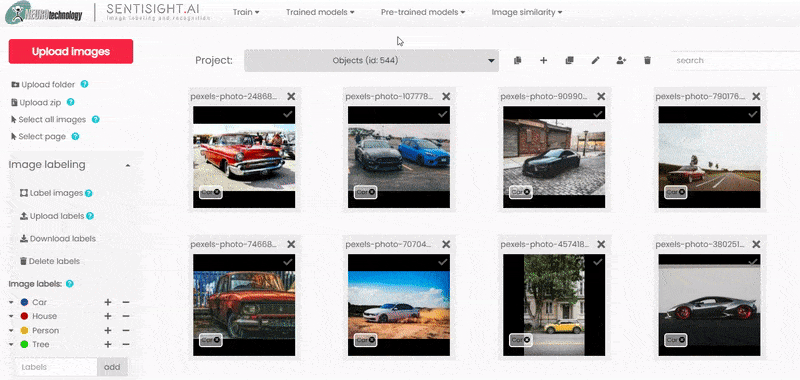
Using pre-trained models via SentiSight.ai REST API
To begin using a pre-trained model via REST API you will need these details:
- API token (available under "User profile" menu tab)
- Model name (either "General-classification", "Places-classification", "NSFW-classification', "Object-detection", "Instance-segmentation", "Text-recognition", "Pose-estimation" or "Background-removal")
Use this endpoint for predictions: https://platform.sentisight.ai/api/pm-predict/{your_model_name}/
A detailed description of the API can be found here.
If you are using the Text recognition model, you also need to add "lang" parameter to the URL (see the section below)
Classification, Object detection, Instance segmentation and Pose estimation samples
Using an image from your computer
Set the "X-Auth-token" header to your API token string and set "Content-Type" header to "application/octet-stream". Set the body to your image file.
For more details, see the code samples below.
TOKEN="your_token"
MODEL="your_model_name" # "General-classification", "Places-classification", "NSFW-classification" or "Object-detection"
IMAGE_FILENAME="your_image_path"
curl -H "X-Auth-token: $TOKEN" --data-binary @"$IMAGE_FILENAME"
-H "Content-Type: application/octet-stream"
-X POST "https://platform.sentisight.ai/api/pm-predict/$MODEL"
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.file.Files;
public class App
{
public static void main( String[] args ) throws IOException
{
if (args.length < 4) {
System.out.println("Usage: java -jar sample.jar api_token file");
}
String token = args[0];
String imageFilename = args[1];
String modelName = "General-classification"; // Change to "General-classification", "Places-classification", "NSFW-classification", "Object-detection" or "Instance-segmentation"
byte[] bytes = Files.readAllBytes(new File(imageFilename).toPath());
URL url = new URL("https://platform.sentisight.ai/api/pm-predict/" + modelName);
HttpURLConnection connection = (HttpURLConnection)url.openConnection();
connection.setRequestProperty("Content-Type", "application/octet-stream");
connection.setRequestProperty("X-Auth-token", token);
connection.setRequestMethod("POST");
connection.setDoOutput(true);
DataOutputStream wr = new DataOutputStream(connection.getOutputStream());
wr.write(bytes);
wr.flush();
wr.close();
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String output;
StringBuffer response = new StringBuffer();
while ((output = in.readLine()) != null) {
System.out.println(output);
response.append(output);
}
in.close();
}
}
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Sample</title>
<script type="text/javascript">
const baseApiURL = 'https://platform.sentisight.ai/api/';
let token = '';
let predictionId;
let results;
let resultOutput;
function predict() {
token = document.getElementById('tokenfield').value;
const modelName = "General-classification" // Change to "General-classification", "Places-classification", "NSFW-classification" or "Object-detection"
const input = document.getElementById('upload');
resultOutput = document.getElementById('output');
const file = input.files[0];
const fr = new FileReader();
fr.onload = function() {
results = apiPostRequest('pm-predict/' + modelName, fr.result);
let parsedResults = JSON.parse(results);
resultOutput.innerText = results
console.log(parsedResults);
}
fr.readAsArrayBuffer(file);
}
function apiPostRequest(request, body) {
const xmlHttp = new XMLHttpRequest();
xmlHttp.open( "POST", baseApiURL + request, false );
xmlHttp.setRequestHeader('Content-Type', 'application/octet-stream');
xmlHttp.setRequestHeader('X-Auth-token', token);
xmlHttp.send(body);
console.log(xmlHttp.responseText);
return xmlHttp.responseText;
}
</script>
</head>
<body>
Token: <input id="tokenfield" type="text" name="" value="">
<br>
Upload image: <input id="upload" type="file" name="" value="">
<br>
<button type="button" onclick="predict()">Predict</button>
<br><br><br>
<p id="output">Your results will go here!</p>
</body>
</html>
import requests
token = "your_token"
model = "your_model_name" # "General-classification", "Places-classification", "NSFW-classification" or "Object-detection"
image_filename = "your_image_path"
headers = {"X-Auth-token": token, "Content-Type": "application/octet-stream"}
with open(image_filename, 'rb') as handle:
r = requests.post('https://platform.sentisight.ai/api/pm-predict/{}/'.format(model), headers=headers, data=handle)
if r.status_code == 200:
print(r.text)
else:
print('Error occured with REST API.')
print('Status code: {}'.format(r.status_code))
print('Error message: ' + r.text)
using System;
using System.IO;
using System.Net.Http;
using System.Net.Http.Headers;
namespace Sample
{
class Program
{
static void Main(string[] args)
{
if (args.Length < 3)
{
Console.WriteLine("Expected arguments: api_token model_name file");
return;
}
var token = args[0];
var modelName = args[1];
var imageFilename = args[2];
var bytes = File.ReadAllBytes(imageFilename);
var data = new ByteArrayContent(bytes);
data.Headers.ContentType = MediaTypeHeaderValue.Parse("application/octet-stream");
var uri = new Uri($"https://platform.sentisight.ai/api/pm-predict/{modelName}");
var client = new HttpClient();
client.DefaultRequestHeaders.Add("X-Auth-token", token);
var response = client.PostAsync(uri, data);
var result = response.Result.Content.ReadAsStringAsync().Result;
Console.WriteLine(result);
}
}
}
Using an image URL
Using the REST API by providing an image URL is similar to the previous case of using REST API by uploading an image. The only differences are that you need to set the "Content-Type" header to "application/json" and set the body to a JSON formatted string with a "url" parameter specifying the image URL.
For more details, see the code samples below.
TOKEN="your_token"
MODEL="your_model_name" # "General-classification", "Places-classification", "NSFW-classification", "Object-detection" or "Pose-estimation"
IMAGE_URL="your_image_url"
curl --location --request POST "https://platform.sentisight.ai/api/pm-predict/$MODEL"
--header 'X-Auth-token: $TOKEN'
--header 'Content-Type: application/json'
--data-raw '{
"url": ""$IMAGE_URL""
}'
package sentisight.api.sample;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class App
{
public static void main( String[] args ) throws IOException
{
String token = "";
String modelName = "";
String image_url = "";
String body = "{rn "url": "%s"rn}".formatted(image_url);
URL url = new URL("https://platform.sentisight.ai/api/pm-predict/" + modelName);
HttpURLConnection connection = (HttpURLConnection)url.openConnection();
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("X-Auth-token", token);
connection.setRequestMethod("POST");
connection.setDoOutput(true);
DataOutputStream wr = new DataOutputStream(connection.getOutputStream());
wr.writeBytes(body);
wr.flush();
wr.close();
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String output;
StringBuilder response = new StringBuilder();
while ((output = in.readLine()) != null) {
response.append(output);
}
System.out.println(response);
System.out.println(connection.getResponseCode());
in.close();
}
}
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Sample</title>
<script type="text/javascript">
const baseApiURL = 'https://platform.sentisight.ai/api/';
let token = '';
let results;
let resultOutput;
function predict() {
token = document.getElementById('tokenfield').value;
resultOutput = document.getElementById('output');
const modelName = "General-classification" // Change to "General-classification", "Places-classification", "NSFW-classification" or "Object-detection"
const url = document.getElementById('url').value;
const data = JSON.stringify({
url
});
results = apiPostRequest('pm-predict/' + modelName, data);
let parsedResults = JSON.parse(results);
resultOutput.innerText = results
console.log(parsedResults);
}
function apiPostRequest(request, body) {
var xmlHttp = new XMLHttpRequest();
xmlHttp.open( "POST", baseApiURL + request, false );
xmlHttp.setRequestHeader('Content-Type', 'application/json');
xmlHttp.setRequestHeader('X-Auth-token', token);
xmlHttp.send(body);
console.log(xmlHttp.responseText);
return xmlHttp.responseText;
}
</script>
</head>
<body>
Token: <input id="tokenfield" type="text" name="" value="">
<br>
Url: <input id="url" type="text" name="" value="">
<br>
<button type="button" onclick="predict()">Predict</button>
<br><br><br>
<p id="output">Your results will go here!</p>
</body>
</html>
import requests
import json
token = "your_token"
model = "your_model_name" # "General-classification", "Places-classification", "NSFW-classification" or "Object-detection"
image_url = "http://your-image-url.png"
payload = json.dumps({
"url": image_url
})
headers = {"X-Auth-token": token, "Content-Type": "application/json"}
r = requests.post('https://platform.sentisight.ai/api/pm-predict/{}/'.format(model), headers=headers, data=payload)
if r.status_code == 200:
print(r.text)
else:
print('Error occured with REST API.')
print('Status code: {}'.format(r.status_code))
print('Error message: ' + r.text)
using System;
using System.IO;
using System.Net.Http;
using System.Text;
using System.Text.Json;
namespace Sample
{
class Program
{
static void Main(string[] args)
{
if (args.Length < 3)
{
Console.WriteLine("Expected arguments: api_token model_name url");
return;
}
var token = args[0];
var modelName = args[1];
var imageUrl = args[2];
using var ms = new MemoryStream();
using var writer = new Utf8JsonWriter(ms);
writer.WriteStartObject();
writer.WriteString("url", imageUrl);
writer.WriteEndObject();
writer.Flush();
string json = Encoding.UTF8.GetString(ms.ToArray());
var data = new StringContent(json, Encoding.Default, "application/json");
var uri = new Uri($"https://platform.sentisight.ai/api/pm-predict/{modelName}");
var client = new HttpClient();
client.DefaultRequestHeaders.Add("X-Auth-token", token);
var response = client.PostAsync(uri, data);
var result = response.Result.Content.ReadAsStringAsync().Result;
Console.WriteLine(result);
}
}
}
Using a Base64 encoded image
Using the REST API by providing a Base64 encoded image is very similar to the case of using REST API with an image URL. The only difference is that you need to change the JSON parameter name "url" to "base64".
For more details, see the code samples below.
TOKEN="your_token"
MODEL="your_model_name" # "General-classification", "Places-classification", "NSFW-classification", "Object-detection" or "Pose-estimation"
IMAGE_B64=""
curl --location --request POST "https://platform.sentisight.ai/api/pm-predict/$MODEL"
--header 'X-Auth-token: $TOKEN'
--header 'Content-Type: application/json'
--data-raw '{
"base64": ""$IMAGE_B64""
}'
package sentisight.api.sample;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class App
{
public static void main( String[] args ) throws IOException
{
String token = "";
String modelName = "";
String image_b64 = "";
String body = "{rn "base64": "%s"rn}".formatted(image_b64);
URL url = new URL("https://platform.sentisight.ai/api/pm-predict/" + modelName);
HttpURLConnection connection = (HttpURLConnection)url.openConnection();
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("X-Auth-token", token);
connection.setRequestMethod("POST");
connection.setDoOutput(true);
DataOutputStream wr = new DataOutputStream(connection.getOutputStream());
wr.writeBytes(body);
wr.flush();
wr.close();
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String output;
StringBuilder response = new StringBuilder();
while ((output = in.readLine()) != null) {
response.append(output);
}
System.out.println(response);
System.out.println(connection.getResponseCode());
in.close();
}
}
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Sample</title>
<script type="text/javascript">
const baseApiURL = 'https://platform.sentisight.ai/api/';
let token = '';
let results;
let resultOutput;
function predict() {
token = document.getElementById('tokenfield').value;
resultOutput = document.getElementById('output');
const modelName = "General-classification" // Change to "General-classification", "Places-classification", "NSFW-classification" or "Object-detection"
const base64 = document.getElementById('base64').value;
const data = JSON.stringify({
base64
});
results = apiPostRequest('pm-predict/' + modelName, data);
let parsedResults = JSON.parse(results);
resultOutput.innerText = results
console.log(parsedResults);
}
function apiPostRequest(request, body) {
var xmlHttp = new XMLHttpRequest();
xmlHttp.open( "POST", baseApiURL + request, false );
xmlHttp.setRequestHeader('Content-Type', 'application/json');
xmlHttp.setRequestHeader('X-Auth-token', token);
xmlHttp.send(body);
console.log(xmlHttp.responseText);
return xmlHttp.responseText;
}
</script>
</head>
<body>
Token: <input id="tokenfield" type="text" name="" value="">
<br>
Base64: <input id="base64" type="text" name="" value="">
<br>
<button type="button" onclick="predict()">Predict</button>
<br><br><br>
<p id="output">Your results will go here!</p>
</body>
</html>
import requests
import json
token = "your_token"
model = "your_model_name" # "General-classification", "Places-classification", "NSFW-classification" or "Object-detection"
image_b64 = ""
payload = json.dumps({
"base64": image_b64
})
headers = {"X-Auth-token": token, "Content-Type": "application/json"}
r = requests.post('https://platform.sentisight.ai/api/pm-predict/{}/'.format(model), headers=headers, data=payload)
if r.status_code == 200:
print(r.text)
else:
print('Error occured with REST API.')
print('Status code: {}'.format(r.status_code))
print('Error message: ' + r.text)
using System;
using System.IO;
using System.Net.Http;
using System.Text;
using System.Text.Json;
namespace Sample
{
class Program
{
static void Main(string[] args)
{
if (args.Length < 4)
{
Console.WriteLine("Expected arguments: api_token project_id model_name base64");
return;
}
var token = args[0];
var projectId = args[1];
var modelName = args[2];
var imageB64 = args[3];
using var ms = new MemoryStream();
using var writer = new Utf8JsonWriter(ms);
writer.WriteStartObject();
writer.WriteString("base64", imageB64);
writer.WriteEndObject();
writer.Flush();
var json = Encoding.UTF8.GetString(ms.ToArray());
var data = new StringContent(json, Encoding.Default, "application/json");
var uri = new Uri($"https://platform.sentisight.ai/api/predict/{projectId}/{modelName}");
var client = new HttpClient();
client.DefaultRequestHeaders.Add("X-Auth-token", token);
var response = client.PostAsync(uri, data);
var result = response.Result.Content.ReadAsStringAsync().Result;
Console.WriteLine(result);
}
}
}
Text recognition samples
Using the text recognition (sometimes also called "Optical Character Recognition" or "OCR") model is very similar to the other models, the main difference is that you need to add "lang" parameter to the URL. Specify the code names of the languages you want to use, separating them with a comma (see code samples below). You can find the supported languages and their codes here. We recommend using English together with other languages as some of its characters often appear in the foreign text.
The REST API query will return a list of the detected text pieces with their confidence scores. To get a continuous parsed text you can simply join the results sequentially.
Below you will find image upload samples for text recognition. You can adapt other pre-trained models' samples using URL or Base64 image to use with text recognition, by adding ?lang=$ALLOWED_LANGUAGES query parameter to the URL.
TOKEN="your_token"
MODEL="Text-recognition"
IMAGE_FILENAME="your_image_path"
ALLOWED_LANGUAGES="de,en"
curl -H "X-Auth-token: $TOKEN" --data-binary @"$IMAGE_FILENAME"
-H "Content-Type: application/octet-stream"
-X POST "https://platform.sentisight.ai/api/pm-predict/$MODEL?lang=$ALLOWED_LANGUAGES"
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.file.Files;
public class App
{
public static void main( String[] args ) throws IOException
{
String modelName = "Text-recognition";
String allowedLanguages="de,en";
byte[] bytes = Files.readAllBytes(new File(imageFilename).toPath());
URL url = new URL("https://platform.sentisight.ai/api/pm-predict/" + modelName +"?lang=" + allowedLanguages);
HttpURLConnection connection = (HttpURLConnection)url.openConnection();
connection.setRequestProperty("Content-Type", "application/octet-stream");
connection.setRequestProperty("X-Auth-token", token);
connection.setRequestMethod("POST");
connection.setDoOutput(true);
DataOutputStream wr = new DataOutputStream(connection.getOutputStream());
wr.write(bytes);
wr.flush();
wr.close();
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String output;
StringBuffer response = new StringBuffer();
while ((output = in.readLine()) != null) {
System.out.println(output);
response.append(output);
}
in.close();
}
}
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Sample</title>
<script type="text/javascript">
const baseApiURL = 'https://platform.sentisight.ai/api/';
let token = '';
let allowedLanguages='de,en';
let predictionId;
let results;
let resultOutput;
function predict() {
token = document.getElementById('tokenfield').value;
const modelName = "Text-recognition" // Change to "General-classification", "Places-classification", "NSFW-classification" or "Object-detection"
const input = document.getElementById('upload');
resultOutput = document.getElementById('output');
const file = input.files[0];
const fr = new FileReader();
fr.onload = function() {
results = apiPostRequest('pm-predict/' + modelName + '?lang=' + allowedLanguages, fr.result);
let parsedResults = JSON.parse(results);
resultOutput.innerText = results
console.log(parsedResults);
}
fr.readAsArrayBuffer(file);
}
function apiPostRequest(request, body) {
const xmlHttp = new XMLHttpRequest();
xmlHttp.open( "POST", baseApiURL + request, false );
xmlHttp.setRequestHeader('Content-Type', 'application/octet-stream');
xmlHttp.setRequestHeader('X-Auth-token', token);
xmlHttp.send(body);
console.log(xmlHttp.responseText);
return xmlHttp.responseText;
}
</script>
</head>
<body>
Token: <input id="tokenfield" type="text" name="" value="">
<br>
Upload image: <input id="upload" type="file" name="" value="">
<br>
<button type="button" onclick="predict()">Predict</button>
<br><br><br>
<p id="output">Your results will go here!</p>
</body>
</html>
import requests
token = "your_token"
model = "Text-recognition"
image_filename = "your_image_path"
allowed_languages="de,en"
headers = {"X-Auth-token": token, "Content-Type": "application/octet-stream"}
with open(image_filename, 'rb') as handle:
r = requests.post('https://platform.sentisight.ai/api/pm-predict/{}?lang={}'.format(model, allowed_languages), headers=headers, data=handle)
if r.status_code == 200:
print(r.text)
else:
print('Error occured with REST API.')
print('Status code: {}'.format(r.status_code))
print('Error message: ' + r.text)
using System;
using System.IO;
using System.Net.Http;
using System.Net.Http.Headers;
namespace Sample
{
class Program
{
static void Main(string[] args)
{
if (args.Length < 3)
{
Console.WriteLine("Expected arguments: api_token allowed_languages file");
return;
}
var token = args[0];
var allowedLanguages = args[1];
var imageFilename= args[2];
const string modelName = "Text-recognition";
var bytes = File.ReadAllBytes(imageFilename);
var data = new ByteArrayContent(bytes);
data.Headers.ContentType = MediaTypeHeaderValue.Parse("application/octet-stream");
var uri = new Uri($"https://platform.sentisight.ai/api/pm-predict/{modelName}?lang={allowedLanguages}");
var client = new HttpClient();
client.DefaultRequestHeaders.Add("X-Auth-token", token);
var response = client.PostAsync(uri, data);
var result = response.Result.Content.ReadAsStringAsync().Result;
Console.WriteLine(result);
}
}
}
Background removal samples
The background removal model is used just like the other models.
The REST API query will return a JSON response which contains base64 encoded PNG image data that can be converted into PNG image file with a variety of apps and online services, such as this.
If you want to trim empty space from the image after removing the background, you can add the "crop=true" parameter to the request URL.
Below you will find image upload samples for background removal. You can adapt other pre-trained models' samples using URL or Base64 image to use with background removal.
TOKEN='your_token'
IMAGE_FILENAME='your_image_path'
curl --location --request POST 'https://platform.sentisight.ai/api/pm-predict/Background-removal' -H 'X-Auth-token: '$TOKEN -H 'Content-Type: application/octet-stream' --data-binary "@$IMAGE_FILENAME"
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.file.Files;
public class App {
public static void main(String[] args) throws IOException {
if (args.length < 4) {
System.out.println("Usage: java -jar sample.jar api_token file");
}
String token = "your_token";
String imageFilename = "your_image_path";
byte[] bytes = Files.readAllBytes(new File(imageFilename).toPath());
URL url = new URL("https://platform.sentisight.ai/api/pm-predict/Background-removal");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestProperty("Content-Type", "application/octet-stream");
connection.setRequestProperty("X-Auth-token", token);
connection.setRequestMethod("POST");
connection.setDoOutput(true);
DataOutputStream wr = new DataOutputStream(connection.getOutputStream());
wr.write(bytes);
wr.flush();
wr.close();
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String output;
StringBuffer response = new StringBuffer();
while ((output = in.readLine()) != null) {
System.out.println(output);
response.append(output);
}
in.close();
}
}
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Sample</title>
<script type="text/javascript">
const baseApiURL = 'https://platform.sentisight.ai/api/pm-predict/Background-removal';
let token = '';
let predictionId;
let results;
let resultOutput;
function predict() {
token = document.getElementById('tokenfield').value;
const input = document.getElementById('upload');
resultOutput = document.getElementById('output');
const file = input.files[0];
const fr = new FileReader();
fr.onload = function() {
results = apiPostRequest(baseApiURL, fr.result);
let parsedResults = JSON.parse(results)[0].image;
resultOutput.innerText = results
console.log(parsedResults);
document.getElementById('output').src = 'data:image/png;base64,' + parsedResults;
}
fr.readAsArrayBuffer(file);
}
function apiPostRequest(request, body) {
const xmlHttp = new XMLHttpRequest();
xmlHttp.open( "POST", request, false );
xmlHttp.setRequestHeader('Content-Type', 'application/octet-stream');
xmlHttp.setRequestHeader('X-Auth-token', token);
xmlHttp.send(body);
console.log(xmlHttp.responseText);
return xmlHttp.responseText;
}
</script>
</head>
<body>
Token: <input id="tokenfield" type="text" name="" value="">
<br>
Upload image: <input id="upload" type="file" name="" value="">
<br>
<button type="button" onclick="predict()">Predict</button>
<br><br><br>
<img id="output">Your results will go here! <img/>
</body>
</html>
import requests
import os
token = "your_token"
image_filename = "your_image_path"
print(image_filename)
headers = {"X-Auth-token": token, "Content-Type": "application/octet-stream"}
with open(image_filename, 'rb') as handle:
r = requests.post('https://platform.sentisight.ai/api/pm-predict/Background-removal', headers=headers, data=handle)
if r.status_code == 200:
print(r.text)
else:
print('Error occured with REST API.')
print('Status code: {}'.format(r.status_code))
print('Error message: ' + r.text)
using System;
using System.IO;
using System.Net.Http;
using System.Net.Http.Headers;
namespace Sample
{
class Program
{
static void Main(string[] args)
{
if (args.Length < 2)
{
Console.WriteLine("Expected arguments: api_token file");
return;
}
var token = args[0];
var imageFilename = args[1];
var modelName = "Background-removal";
var bytes = File.ReadAllBytes(imageFilename);
var data = new ByteArrayContent(bytes);
data.Headers.ContentType = MediaTypeHeaderValue.Parse("application/octet-stream");
var uri = new Uri($"https://platform.sentisight.ai/rs/api/pm-predict/{modelName}");
var client = new HttpClient();
client.DefaultRequestHeaders.Add("X-Auth-token", token);
var response = client.PostAsync(uri, data);
var result = response.Result.Content.ReadAsStringAsync().Result;
Console.WriteLine(result);
}
}
}
SentiSight.ai OpenAPI specification
List of endpoints: https://app.swaggerhub.com/apis-docs/SentiSight.ai/sentisight.
API code samples: https://app.swaggerhub.com/apis/SentiSight.ai/sentisight.
You can try out our REST API interactively and convert Swagger specification to code samples in many different languages.
Using the model offline—setting up your own REST API server
For a variety of reasons you might want to use a trained model independently from SentiSight.ai platform, or indeed without internet connection at all. You can do that by setting up your own REST API server with a model you trained on SentiSight.ai.
In order to set up your own REST API server, you will have to download an offline version of the model. To do that, open Pre-trained model dropdown in the main dashboard and click on the button to download the desired model.
After the model is downloaded, follow the instructions in README.html to set up your local REST API server. You can make the client requests from the same PC on which you set up the server, so the model would be run completely offline. On the other hand, after you set up REST API server, you can also make client requests to this server from many different devices (including mobile) on your network. Note that the REST API server must be run on a Linux system, but the client devices can run on any operating system.
The offline version of the model can be run as a free trial for 30 days. After this period, if you like the offline version, you will have to buy a license from us. If you would like to purchase offline model license, please contact us.
Contents
- Using pre-trained models
- Using pre-trained models via SentiSight.ai web platform
- Using pre-trained models via SentiSight.ai REST API