How it works
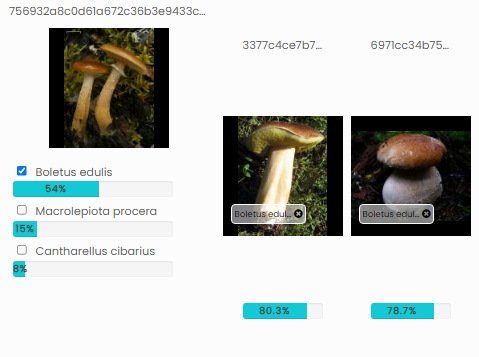
Labeling by Similarity directly compares images to already labeled images and assigns labels based on how similar they are.
Labeling by Similarity can be used right off the bat, without training a model - all you need are some labeled images in your project.
It works as follows:
- Upload images.
- Label some of those images by hand.
- Use Labeling by similarity to automatically label the rest of the images.
Labeling by Similarity tool can either be used to assist with image labeling or as an image classification prediction tool.
You can perform label by similarity requests on your images using the platform or via REST API.
Use cases
AI-assisted and iterative labeling
Label by similarity can simply be used to assist your image labeling process for classification. Instead of labeling all images yourself, you can first label just a handful of images and the use "Label by Similarity tool" to get suggested labels for the rest of unlabeled images. Reviewing suggested labels is typically faster than labeling the images from scratch, so it speeds up your labeling process. Compared to traditional AI-assisted labeling where you usually need to train a classification or object detection model to get assisted labels, the Label by Similirity feature has an additional advantage that no model needs to be trained.
Another advantage of Label by Similarity tool is that you can use it for iterative labeling. The iterative labeling process goes as follows. First you need to label one batch of images by hand. Then you can use Label by Similarity tool to get the suggested labels for a second batch of images. Afterwards, you can review those suggested labels and correct them if necessary. Next, you can use Label by Similarity to get label suggestions for the third batch of images. The suggestions for the third batch of images will be more accurate than for the second batch, because Label by Similarity tool will use both images from the first and the second batch to suggest labels for the images from the third batch. After you finish reviewing labeling images from the third batch, you can proceed with the fourth, the fifth batch, and so on. The more labeled images there are, the more accurate suggested labels will be. So the labeling process will speed up gradually, because you will need to correct less labels while reviewing, if any.
How many images should be in a single batch is up for the user to decide. We do not have a suggested number, but typically, something between 10 to 100 images should work fine. You can either upload all images you have and then use Label by Similarity tool on already uploaded images by selecting the batch of already uploaded images or you can upload the images batch by batch and use the Label by Similarity tool while uploading. There is no difference in terms of an algorithm, so the user can choose which method is more convenient for them.
Classification predictions
Interestingly, Label by Similarity can also be used instead of classification model training, to quickly generate predictions with minimal time input or number of images required. While not always as accurate as predictions made on a properly trained classification model, Label by Similarity will offer you predictions even if you have just one image per label, and it won't demand time to train a model either.
Using Label by Similarity instead of classification model makes most sense when you are using the model via the REST API. You can first use the web platform interface to get a feeling which parameters ("Number of results" and "Threshold") work best in your case and then you can make REST API requests for Label by Similarity using those parameters. See Labeling by similarity using for code samples.
Uploading images
If you are using the platform, press the red Upload button to upload individual images, or Upload folder or Upload zip buttons to upload large numbers of images.
If you are planning to use the API for image upload, read more about it here.
Note that labeling by similarity becomes more accurate the more labeled images you have in your project.
Labeling by similarity using web platform
To use Labeling by similarity in web platform, go to Image Similarity - Label by Similarity and upload images you want to label.
You can also use Label by similarity to assign labels to images already in the project. To do so, just select the images you want to be labeled, right click on them and click AI Tools - Label by similarity.
You will see your query images, a selection of the most similar existing images for each query, as well as labels assigned to the query images, which you will be able to review and edit.
In similarity labeling window you can change the number of images that each image will be compared to, as well as similarity threshold necessary for automatic labeling.
- Number of results - how many already labeled images will each uploaded image be compared to.
- Mark only top scoring label - only one label will be automatically ticked, useful for single-label classification training.
- Threshold - similarity threshold, below which labels will not be automatically assigned.
The default values for "Number of results" is 10 and for "Threshold" is 30%. However, we suggest to try to play around with these parameters and see what works best for your data. For example, in some cases, setting "Number of results" to just 1 or 2 can give better results then the default value (10), especially in cases when you have very few images labeled for each class.
You can also assign or remove suggested labels, allowing you to correct inaccurate predictions.
Please note that changing any parameters will discard any manually applied labels. So we suggest tuning the parameters before starting to make any manual changes to the labels.
After you are done adjusting values and assigning labels, don't forget to click Add to dataset to save your changes and add any new images to your project.
Labeling by similarity using REST API
You can also use similarity labeling via REST API.
Label by similarity using an image from your computer
Set the "X-Auth-token" header to your API token string and set "Content-Type" header to "application/octet-stream". Set the body to your image file.
For more details, see the code samples below.
Using image on your computer
TOKEN="your_token"
PROJECT_ID="your_project_id"
IMAGE_FILENAME="your_image_name" # This is the filepath to the image on your PC
SAVE="false"
USE_FIRST="false"
THRESHOLD="10"
curl --location --request POST 'http://localhost:9080/rs/api/similarity-labels?project=1414&threshold=10&save=true&use-first=true'
--header 'X-Auth-token: m4go96jsof5jhmq820kncfjln5'
--header 'Content-Type: application/octet-stream'
--data-binary '@$IMAGE_FILENAME'
package sentisight.api.sample;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.file.Files;
public class App {
public static void main(String[] args) throws IOException {
String token = "your_token";
String projectId = "your_project_id";
int threshold = 10;
String imageFilename = "your_image_path";
StringBuilder paramsString = new StringBuilder();
paramsString.append("&save=false"); // to add results to dataset, use '&save=true'
paramsString.append("&use-first=false"); // uses results with scores above threshold.
// To get only the result with the highest score, use '&use-first=true'
paramsString.append("&threshold=").append(threshold);
URL url = new URL("https://platform.sentisight.ai/api/similarity-labels?project=" + projectId + paramsString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestProperty("Content-Type", "application/octet-stream");
connection.setRequestProperty("X-Auth-token", token);
connection.setRequestMethod("POST");
connection.setDoOutput(true);
DataOutputStream wr = new DataOutputStream(connection.getOutputStream());
byte[] bytes = Files.readAllBytes(new File(imageFilename).toPath());
wr.write(bytes);
wr.flush();
wr.close();
InputStream stream = connection.getInputStream();
BufferedReader in = new BufferedReader(new InputStreamReader(stream));
String output;
while ((output = in.readLine()) != null) {
System.out.println(output);
}
in.close();
}
}
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Sample</title>
<script>
const baseApiURL = 'https://platform.sentisight.ai/api/';
function similaritySearch() {
document.getElementById('button').disabled = true;
var input = document.getElementById('upload');
const file = input.files[0];
var fr = new FileReader();
fr.onload = function() {
performSimilaritySearch(fr.result);
}
if (file) {
fr.readAsArrayBuffer(file);
}
}
function performSimilaritySearch(file) {
var project = document.getElementById('project').value;
var token = document.getElementById('token').value;
var threshold = document.getElementById('threshold').value;
var save = document.getElementById('save').checked;
var first = document.getElementById('first').checked;
var xmlHttp = new XMLHttpRequest();
xmlHttp.open('POST', baseApiURL + 'similarity-labels?project=' + project + "&threshold=" + threshold + "&save=" + save + "&use-first=" + first, true);
xmlHttp.setRequestHeader('Content-Type', 'application/octet-stream');
xmlHttp.setRequestHeader('X-Auth-token', token);
xmlHttp.onreadystatechange = function () {
if (this.readyState == 4 && this.status == 200) {
document.getElementById('button').disabled = false;
document.getElementById('results').innerHTML = xmlHttp.responseText;
}
}
xmlHttp.send(file);
}
</script>
</head>
<body>
Token: <input id="token" type="text">
<br>
Project id: <input id="project" type="number">
<br>
Threshold: <input id="threshold" type="number">
<br>
Save results: <input id="save" type="checkbox">
<br>
Use first result: <input id="first" type="checkbox">
<br>
Upload image: <input id="upload" type="file">
<br>
<button id="button" type="button" onclick="similaritySearch()">Perform similarity search</button>
<br>
<table id=results></table>
</body>
</html>
import requests
token = "your_token"
project_id = "your_project_id"
image_filename = "your_image_path"
threshold = 10 # only uses results above this threshold (value in percent)
first = False
save = False
headers = {"X-Auth-token": token, "Content-Type": "application/octet-stream"}
request_url = "https://platform.sentisight.ai/api/similarity-labels?project={}".format(project_id)
request_url += "&save={}".format(save) # to add results to dataset, use '&save=true'
request_url += "&use-first={}".format(first) # uses results with scores above threshold.
# To get only the result with the highest score, use '&use-first=true'
request_url += "&threshold={}".format(threshold) # unused if 'use-first' is true
with open(image_filename, "rb") as handle:
r = requests.post(request_url, headers=headers, data=handle)
if r.status_code == 200:
print(r.text)
else:
print("Error occured with REST API.")
print("Status code: {}".format(r.status_code))
print("Error message: " + r.text)
using System;
using System.IO;
using System.Linq;
using System.Net.Http;
using System.Net.Http.Headers;
namespace Sample
{
class Program
{
static void Main()
{
const string token = "";
const int projectId = 0;
const string imageFilename = "";
const double threshold = 10;
var paramString = "";
paramString += ("&save=false");
paramString += ("&use-first=false");
paramString += ("&threshold=" + threshold);
var bytes = File.ReadAllBytes(imageFilename);
var data = new ByteArrayContent(bytes);
data.Headers.ContentType = MediaTypeHeaderValue.Parse("application/octet-stream");
var uri = new Uri($"http://platform.sentisight.ai/api/similarity-labeling?project={projectId}{paramString}");
var client = new HttpClient();
client.DefaultRequestHeaders.Add("X-Auth-token", token);
var response = client.PostAsync(uri, data);
var result = response.Result.Content.ReadAsStringAsync().Result;
Console.WriteLine(result);
}
}
}
Label by similarity using an image URL
Labeling by similarity via REST API using an image URL is similar to the previous case of uploading an image. The only differences are that you need to set the "Content-Type" header to "application/json" and set the body to a JSON formatted string with a "url" parameter specifying the image URL.
For more details, see the code samples below.
Using image URL
TOKEN="your_token"
PROJECT_ID="your_project_id"
IMAGE_FILENAME="your_image_name" # This is the filepath to the image on your PC
SAVE="false"
USE_FIRST="false"
THRESHOLD="10"
curl --location --request POST 'http://localhost:9080/rs/api/similarity-labels?project=1414&threshold=10&save=true&use-first=true'
--header 'X-Auth-token: m4go96jsof5jhmq820kncfjln5'
--header 'Content-Type: application/octet-stream'
--data-binary '@$IMAGE_FILENAME'
package sentisight.api.sample;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class App {
public static void main(String[] args) throws IOException {
String token = "your_token";
String projectId = "your_project_id";
int threshold = 10;
String imageUrl = "";
StringBuilder paramsString = new StringBuilder();
paramsString.append("&save=false"); // to add results to dataset, use '&save=true'
paramsString.append("&use-first=false"); // uses results with scores above threshold.
// To get only the result with the highest score, use '&use-first=true'
paramsString.append("&threshold=").append(threshold);
String body = String.format("{"url": "%s"}", imageUrl);
URL url = new URL("https://platform.sentisight.ai/api/similarity-labels?project=" + projectId + paramsString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("X-Auth-token", token);
connection.setRequestMethod("POST");
connection.setDoOutput(true);
DataOutputStream wr = new DataOutputStream(connection.getOutputStream());
wr.writeBytes(body);
wr.flush();
wr.close();
InputStream stream = connection.getInputStream();
BufferedReader in = new BufferedReader(new InputStreamReader(stream));
String output;
while ((output = in.readLine()) != null) {
System.out.println(output);
}
in.close();
}
}
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Sample</title>
<script>
const baseApiURL = 'https://platform.sentisight.ai/api/';
function similaritySearch() {
var project = document.getElementById('project').value;
var token = document.getElementById('token').value;
var threshold = document.getElementById('threshold').value;
var save = document.getElementById('save').checked;
var first = document.getElementById('first').checked;
var url = document.getElementById('image').value;
var data = JSON.stringify({
url
});
var xmlHttp = new XMLHttpRequest();
xmlHttp.open('POST', baseApiURL + 'similarity-labels?project=' + project + "&threshold=" + threshold + "&save=" + save + "&use-first=" + first, true);
xmlHttp.setRequestHeader('Content-Type', 'text/plain');
xmlHttp.setRequestHeader('X-Auth-token', token);
xmlHttp.onreadystatechange = function () {
if (this.readyState == 4 && this.status == 200) {
document.getElementById('button').disabled = false;
document.getElementById('results').innerHTML = xmlHttp.responseText;
}
}
xmlHttp.send(data);
}
</script>
</head>
<body>
Token: <input id="token" type="text">
<br>
Project id: <input id="project" type="number">
<br>
Threshold: <input id="threshold" type="number">
<br>
Save results: <input id="save" type="checkbox">
<br>
Use first result: <input id="first" type="checkbox">
<br>
Image URL: <input id="image" type="text">
<br>
<button id="button" type="button" onclick="similaritySearch()">Perform similarity search</button>
<br>
<table id=results></table>
</body>
</html>
import requests
import json
token = "your_token"
project_id = "your_project_id"
image_url = "your_image_url"
threshold = 10 # only uses results above this threshold (value in percent)
first = False
payload = json.dumps({
"url": image_url
})
headers = {"X-Auth-token": token, "Content-Type": "application/json"}
request_url = "https://platform.sentisight.ai/api/similarity-labels?project={}".format(project_id)
request_url += "&save=false" # to add results to dataset, use '&save=true'
request_url += "&use-first=false" # uses results with scores above threshold.
# To get only the result with the highest score, use '&use-first=true'
request_url += "&threshold={}".format(threshold) # unused if 'use-first' is true
r = requests.post(request_url, headers=headers, data=payload)
if r.status_code == 200:
print(r.text)
else:
print("Error occured with REST API.")
print("Status code: {}".format(r.status_code))
print("Error message: " + r.text)
using System;
using System.IO;
using System.Linq;
using System.Net.Http;
using System.Net.Http.Headers;
namespace Sample
{
class Program
{
static void Main()
{
const string token = "";
const int projectId = 0;
const string imageUrl = "";
const double threshold = 10;
var paramString = "";
paramString += ("&save=false");
paramString += ("&use-first=false");
paramString += ("&threshold=" + threshold);
using var ms = new MemoryStream();
using var writer = new Utf8JsonWriter(ms);
writer.WriteStartObject();
writer.WriteString("url", imageUrl);
writer.WriteEndObject();
writer.Flush();
var json = Encoding.UTF8.GetString(ms.ToArray());
var data = new StringContent(json, Encoding.Default, "application/json");
var uri = new Uri($"https://platform.sentisight.ai/api/similarity-labels?project={projectId}{paramString}");
var client = new HttpClient();
client.DefaultRequestHeaders.Add("X-Auth-token", token);
var response = client.PostAsync(uri, data);
var result = response.Result.Content.ReadAsStringAsync().Result;
Console.WriteLine(result);
}
}
}
Label by similarity using an image in your project
Set the "X-Auth-token" header to your API token string and set "Content-Type" header to "application/json". Set the body to a JSON formatted string with a "imageName" parameter specifying your query image name. The query image should be already uploaded to the SentiSight.ai platform.
For more details, see the code samples below.
Using an exisiting image from a project
TOKEN="your_token"
PROJECT_ID="your_project_id"
IMAGE_NAME="your_image_name" # this the name of an image which is already uploaded on platform.sentisight.ai
SAVE="false"
USE_FIRST="false"
THRESHOLD="10"
curl --location --request POST 'https://platform.sentisight.ai/api/similarity-labels?project='$PROJECT_ID'&threshold='$THRESHOLD'&save='$SAVE'&use-first='$USE_FIRST
--header 'X-Auth-token: '$TOKEN
--header 'Content-Type: application/json'
--data-raw '{ "imageName": "'$IMAGE_NAME'" }'
package sentisight.api.sample;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class App {
public static void main(String[] args) throws IOException {
String token = "your_token";
String projectId = "your_project_id";
int threshold = 10;
String imageName = "image.jpg";
StringBuilder paramsString = new StringBuilder();
paramsString.append("&save=false"); // to add results to dataset, use '&save=true'
paramsString.append("&use-first=false"); // uses results with scores above threshold.
// To get only the result with the highest score, use '&use-first=true'
paramsString.append("&threshold=").append(threshold);
String body = String.format("{"imageName": "%s"}", imageName);
URL url = new URL("https://platform.sentisight.ai/api/similarity-labels?project=" + projectId + paramsString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("X-Auth-token", token);
connection.setRequestMethod("POST");
connection.setDoOutput(true);
DataOutputStream wr = new DataOutputStream(connection.getOutputStream());
wr.writeBytes(body);
wr.flush();
wr.close();
InputStream stream = connection.getInputStream();
BufferedReader in = new BufferedReader(new InputStreamReader(stream));
String output;
while ((output = in.readLine()) != null) {
System.out.println(output);
}
in.close();
}
}
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Sample</title>
<script>
const baseApiURL = 'https://platform.sentisight.ai/api/';
function similaritySearch() {
var project = document.getElementById('project').value;
var token = document.getElementById('token').value;
var threshold = document.getElementById('threshold').value;
var save = document.getElementById('save').checked;
var first = document.getElementById('first').checked;
var image = document.getElementById('image').value;
var data = JSON.stringify({
imageName: image
});
var xmlHttp = new XMLHttpRequest();
xmlHttp.open('POST', baseApiURL + 'similarity-labels?project=' + project + "&threshold=" + threshold + "&save=" + save + "&use-first=" + first, true);
xmlHttp.setRequestHeader('Content-Type', 'text/plain');
xmlHttp.setRequestHeader('X-Auth-token', token);
xmlHttp.onreadystatechange = function () {
if (this.readyState == 4 && this.status == 200) {
document.getElementById('button').disabled = false;
document.getElementById('results').innerHTML = xmlHttp.responseText;
}
}
xmlHttp.send(data);
}
</script>
</head>
<body>
Token: <input id="token" type="text">
<br>
Project id: <input id="project" type="number">
<br>
Threshold: <input id="threshold" type="number">
<br>
Save results: <input id="save" type="checkbox">
<br>
Use first result: <input id="first" type="checkbox">
<br>
Image name: <input id="image" type="text">
<br>
<button id="button" type="button" onclick="similaritySearch()">Perform similarity search</button>
<br>
<table id=results></table>
</body>
</html>
import requests
import json
token = "your_token"
project_id = "your_project_id"
image_name = "your_image_name"
threshold = 10 # only uses results above this threshold (value in percent)
first = False
payload = json.dumps({
"imageName": image_name
})
headers = {"X-Auth-token": token, "Content-Type": "application/json"}
request_url = "https://platform.sentisight.ai/api/similarity-labels?project={}".format(project_id)
request_url += "&save=false" # to add results to dataset, use '&save=true'
request_url += "&use-first=false" # uses results with scores above threshold.
# To get only the result with the highest score, use '&use-first=true'
request_url += "&threshold={}".format(threshold) # unused if 'use-first' is true
r = requests.post(request_url, headers=headers, data=payload)
if r.status_code == 200:
print(r.text)
else:
print("Error occured with REST API.")
print("Status code: {}".format(r.status_code))
print("Error message: " + r.text)
using System;
using System.IO;
using System.Linq;
using System.Net.Http;
using System.Net.Http.Headers;
namespace Sample
{
class Program
{
static void Main()
{
const string token = "";
const int projectId = 0;
const string imageName = "";
const double threshold = 10;
var paramString = "";
paramString += ("&save=false");
paramString += ("&use-first=false");
paramString += ("&threshold=" + threshold);
using var ms = new MemoryStream();
using var writer = new Utf8JsonWriter(ms);
writer.WriteStartObject();
writer.WriteString("imageName", imageName);
writer.WriteEndObject();
writer.Flush();
var json = Encoding.UTF8.GetString(ms.ToArray());
var data = new StringContent(json, Encoding.Default, "application/json");
var uri = new Uri($"https://platform.sentisight.ai/api/similarity-labels?project={projectId}{paramString}");
var client = new HttpClient();
client.DefaultRequestHeaders.Add("X-Auth-token", token);
var response = client.PostAsync(uri, data);
var result = response.Result.Content.ReadAsStringAsync().Result;
Console.WriteLine(result);
}
}
}
Label by similarity using a Base64 encoded image
Using the REST API by providing a Base64 encoded image is very similar to the case of using REST API with an image URL. The only difference is that you need to change the JSON parameter name "url" to "base64".
For more details, see the code samples below.
Using Base64 image
TOKEN="your_token"
PROJECT_ID="your_project_id"
IMAGE_B64=""
SAVE="false"
USE_FIRST="false"
THRESHOLD="10"
curl --location --request POST 'https://platform.sentisight.ai/api/similarity-labels?project='$PROJECT_ID'&threshold='$THRESHOLD'&save='$SAVE'&use-first='$USE_FIRST
--header 'X-Auth-token: '$TOKEN
--header 'Content-Type: application/json'
--data '{
"base64": ""$IMAGE_B64""
}
package sentisight.api.sample;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class App {
public static void main(String[] args) throws IOException {
String encodstring = null;
try {
encodstring = encoder(""); // image path
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
System.out.println(encodstring);
String token = "your_token";
String projectId = "your_project_id";
int threshold = 10;
String imageB64 = "";
StringBuilder paramsString = new StringBuilder();
paramsString.append("&save=false"); // to add results to dataset, use '&save=true'
paramsString.append("&use-first=false"); // uses results with scores above threshold.
// To get only the result with the highest score, use '&use-first=true'
paramsString.append("&threshold=").append(threshold);
String body = String.format("{"base64": "%s"}", imageB64);
URL url = new URL("https://platform.sentisight.ai/api/similarity-labels?project=" + projectId + paramsString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("X-Auth-token", token);
connection.setRequestMethod("POST");
connection.setDoOutput(true);
DataOutputStream wr = new DataOutputStream(connection.getOutputStream());
wr.writeBytes(body);
wr.flush();
wr.close();
InputStream stream = connection.getInputStream();
BufferedReader in = new BufferedReader(new InputStreamReader(stream));
String output;
while ((output = in.readLine()) != null) {
System.out.println(output);
}
in.close();
}
public static String encoder(String filePath) {
String base64File = "";
File file = new File(filePath);
try (FileInputStream imageInFile = new FileInputStream(file)) {
// Reading a file from file system
byte fileData[] = new byte[(int) file.length()];
imageInFile.read(fileData);
base64File = Base64.getEncoder().encodeToString(fileData);
} catch (FileNotFoundException e) {
System.out.println("File not found" + e);
} catch (IOException ioe) {
System.out.println("Exception while reading the file " + ioe);
}
return base64File;
}
}
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Sample</title>
<script>
const baseApiURL = 'https://platform.sentisight.ai/api/';
function similaritySearch() {
var project = document.getElementById('project').value;
var token = document.getElementById('token').value;
var threshold = document.getElementById('threshold').value;
var save = document.getElementById('save').checked;
var first = document.getElementById('first').checked;
var base64 = document.getElementById('image').value;
var data = JSON.stringify({
base64
});
var xmlHttp = new XMLHttpRequest();
xmlHttp.open('POST', baseApiURL + 'similarity-labels?project=' + project + "&threshold=" + threshold + "&save=" + save + "&use-first=" + first, true);
xmlHttp.setRequestHeader('Content-Type', 'text/plain');
xmlHttp.setRequestHeader('X-Auth-token', token);
xmlHttp.onreadystatechange = function () {
if (this.readyState == 4 && this.status == 200) {
document.getElementById('button').disabled = false;
document.getElementById('results').innerHTML = xmlHttp.responseText;
}
}
xmlHttp.send(data);
}
</script>
</head>
<body>
Token: <input id="token" type="text">
<br>
Project id: <input id="project" type="number">
<br>
Threshold: <input id="threshold" type="number">
<br>
Save results: <input id="save" type="checkbox">
<br>
Use first result: <input id="first" type="checkbox">
<br>
Base64: <input id="image" type="text">
<br>
<button id="button" type="button" onclick="similaritySearch()">Perform similarity search</button>
<br>
<table id=results></table>
</body>
</html>
import requests
import json
import base64
with open("C:\Users\laurynass\Documents\test\8fb2db66f82541410e4f905aa78432428cf1dfa0.jpg", "rb") as image_file:
encoded_string = base64.b64encode(image_file.read()).decode("UTF-8")
token = "your_token"
project_id = "your_project_id"
image_b64 = ""
threshold = 10 # only uses results above this threshold (value in percent)
first = False
payload = json.dumps({
"base64": image_b64
})
headers = {"X-Auth-token": token, "Content-Type": "application/json"}
request_url = "https://platform.sentisight.ai/api/similarity-labels?project={}".format(project_id)
request_url += "&save=false" # to add results to dataset, use '&save=true'
request_url += "&use-first=false" # uses results with scores above threshold.
# To get only the result with the highest score, use '&use-first=true'
request_url += "&threshold={}".format(threshold) # unused if 'use-first' is true
r = requests.post(request_url, headers=headers, data=payload)
if r.status_code == 200:
print(r.text)
else:
print("Error occured with REST API.")
print("Status code: {}".format(r.status_code))
print("Error message: " + r.text)
using System;
using System.IO;
using System.Linq;
using System.Net.Http;
using System.Net.Http.Headers;
namespace Sample
{
class Program
{
static void Main()
{
const string token = "";
const int projectId = 0;
const string base64 = "";
const double threshold = 10;
var paramString = "";
paramString += ("&save=false");
paramString += ("&use-first=false");
paramString += ("&threshold=" + threshold);
using var ms = new MemoryStream();
using var writer = new Utf8JsonWriter(ms);
writer.WriteStartObject();
writer.WriteString("base64", base64);
writer.WriteEndObject();
writer.Flush();
var json = Encoding.UTF8.GetString(ms.ToArray());
var data = new StringContent(json, Encoding.Default, "application/json");
var uri = new Uri($"https://platform.sentisight.ai/api/similarity-labels?project={projectId}{paramString}");
var client = new HttpClient();
client.DefaultRequestHeaders.Add("X-Auth-token", token);
var response = client.PostAsync(uri, data);
var result = response.Result.Content.ReadAsStringAsync().Result;
Console.WriteLine(result);
}
}
}
SentiSight.ai OpenAPI specification
List of endpoints: https://app.swaggerhub.com/apis-docs/SentiSight.ai/sentisight.
API code samples: https://app.swaggerhub.com/apis/SentiSight.ai/sentisight.
You can try out our REST API interactively and convert Swagger specification to code samples in many different languages.
Using Label by Similarity offline
Please note, that currently, we do not have a separate "Label by Similarity" offline model, but users can use our regular "Image similarity" model for those purposes. The best way to do it would be to store the image labels locally and once you get the similarity search results, calculate suggested labels manually. The suggested labels are actually labels of similarity hits multiplied by their similarity scores. See more here.
Video tutorial
For more information, check out the video tutorial detailing labeling by similarity.
Labeling by image similarity tutorial
Topics covered:
- Labeling by image similarity feature
- Changing parameters
- Adjusting suggested labels manually
- Performing AI-assisted labeling iteratively
- Downloading classification labels
Contents
- How it works
- Use cases
- Uploading images
- Labeling by similarity using web platform
- Labeling by similarity using REST API
- Using Label by Similarity offline
- Video tutorial